Greetings,
Creating this tread in hope to create a cheap solution for having a nice image + capturing licence plate without having 2 cameras or an expensive one.
I need testers for various hikvision cameras. I am giving away source + compiled version in return for your feedback.
Please let me know what else would need to be changed or added as a variable inside config file.
All you need to do is update configfile with your data, run exe and trigger linecrossing on camera.(Please install .net runtime 6.0 min, in order to run app)
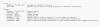
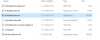
Source:
After testing, please comment out:
Camera Model:
Camera firmware:
Scenario:
Results comment:
Adjustments neded:
Thank you, cheers
Creating this tread in hope to create a cheap solution for having a nice image + capturing licence plate without having 2 cameras or an expensive one.
I need testers for various hikvision cameras. I am giving away source + compiled version in return for your feedback.
Please let me know what else would need to be changed or added as a variable inside config file.
All you need to do is update configfile with your data, run exe and trigger linecrossing on camera.(Please install .net runtime 6.0 min, in order to run app)
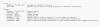
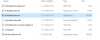
Source:
C#:
using Newtonsoft.Json;
using System;
using System.IO;
using System.Net;
using System.Net.Http.Headers;
using System.Net.Sockets;
using System.Text;
using System.Text.RegularExpressions;
namespace TcpServerExample
{
class Program
{
class Config
{
public ServerConfig Server { get; set; }
public CameraConfig Camera { get; set; }
}
class ServerConfig
{
public string IpAddress { get; set; }
public int Port { get; set; }
}
class CameraConfig
{
public string IpAddress { get; set; }
public string cameraPort { get; set; }
public string Username { get; set; }
public string Password { get; set; }
public string SlowShutter { get; set; }
public string FastShutter { get; set; }
public int switchDelay { get; set; }
}
static void Main(string[] args)
{
/ Load configuration for the server
string jsonConfig = File.ReadAllText("config.json");
Config config = JsonConvert.DeserializeObject<Config>(jsonConfig);
IPAddress serverAddr = IPAddress.Parse(config.Server.IpAddress);
int port = config.Server.Port;
string cameraIp = config.Camera.IpAddress;
string cameraPort = config.Camera.cameraPort;
/ Use camera configuration from JSON
string cameraUsername = config.Camera.Username;
string cameraPassword = config.Camera.Password;
string slowShutter = config.Camera.SlowShutter;
string fastShutter = config.Camera.FastShutter;
int switchDelay = config.Camera.switchDelay;
TcpListener server = new TcpListener(serverAddr, port);
server.Start();
Console.WriteLine($"Server is listening on {cameraIp}:{port}...");
while (true)
{
Console.WriteLine("Waiting for client...");
using (TcpClient client = server.AcceptTcpClient())
{
Console.WriteLine("Client connected.");
try
{
using (NetworkStream stream = client.GetStream())
{
byte[] buffer = new byte[1024]; / Smaller buffer size
int bytesRead;
StringBuilder requestBuilder = new StringBuilder();
while ((bytesRead = stream.Read(buffer, 0, buffer.Length)) > 0)
{
requestBuilder.Append(Encoding.UTF8.GetString(buffer, 0, bytesRead));
/ Check if we have read the linedetection data
if (requestBuilder.ToString().Contains("name=\"linedetection\""))
{
/ Process only the relevant part
HandleLineDetectionEvent(cameraIp,cameraPort,slowShutter,fastShutter, cameraUsername, cameraPassword, switchDelay);
break;
}
}
/ Send a response back to the client
byte[] responseBytes = Encoding.ASCII.GetBytes("HTTP/1.1 200 OK\r\nContent-Length: 0\r\n\r\n");
stream.Write(responseBytes, 0, responseBytes.Length);
}
}
catch (Exception ex)
{
Console.WriteLine($"Exception: {ex.Message}");
}
Console.WriteLine("Client disconnected.");
}
}
}
static async void HandleLineDetectionEvent(string cameraIp, string cameraPort, string slowShutter,string fastShutter,string cameraUsername,string cameraPassword, int switchDelay)
{
string cameraUrl = "http://"+ cameraIp + "/ISAPI/Image/channels/1/shutter";
var credentialCache = new CredentialCache();
credentialCache.Add(new Uri(cameraUrl), "Digest", new NetworkCredential(cameraUsername, cameraPassword));
using var httpClientHandler = new HttpClientHandler
{
Credentials = credentialCache
};
using var httpClient = new HttpClient(httpClientHandler);
/ Set common headers (if necessary, adjust according to your requirements)
httpClient.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("*/*"));
httpClient.DefaultRequestHeaders.Connection.Add("keep-alive");
httpClient.DefaultRequestHeaders.CacheControl = new CacheControlHeaderValue { NoCache = true };
/ First PUT request
var firstContent = new StringContent("<?xml version=\"1.0\" encoding=\"UTF-8\"?><Shutter><ShutterLevel>1/"+fastShutter+"</ShutterLevel></Shutter>", Encoding.UTF8, "application/x-www-form-urlencoded");
var firstResponse = await httpClient.PutAsync(cameraUrl, firstContent);
Console.WriteLine("Switching to fast shutter: " + firstResponse.StatusCode);
/ Wait for 5 seconds
await Task.Delay(switchDelay);
/ Second PUT request
var secondContent = new StringContent("<?xml version=\"1.0\" encoding=\"UTF-8\"?><Shutter><ShutterLevel>1/"+slowShutter+"</ShutterLevel></Shutter>", Encoding.UTF8, "application/x-www-form-urlencoded");
var secondResponse = await httpClient.PutAsync(cameraUrl, secondContent);
Console.WriteLine("Switching back to slow shutter: " + secondResponse.StatusCode);
}
}
}
After testing, please comment out:
Camera Model:
Camera firmware:
Scenario:
Results comment:
Adjustments neded:
Thank you, cheers
Attachments
Last edited: